This article has been archived, as it was published several years ago, so some of its information might now be outdated. For more recent articles, please visit the main article feed.
Multiline string literal tips and tricks
Basics article available: StringsIt’s fair to say that most of the strings that we include within a given project should most likely not be hard-coded within our source code, as doing so prevents us from localizing and working with those strings outside of our code base.
However, there are situations in which we might want to inline even somewhat longer strings within our Swift code, and in those situations multiline string literals can come very much in handy.
For example, if we wanted to define an info message to be printed as part of a command line tool, we could make that happen using a single string literal, like this:
let infoMessage = """
Welcome to my animation tool. You can use it to:
- Convert images into animations.
- Split an animation up into images.
- Change the frame rate of an animation.
"""
We could also indent the above message, as multiline string literals don’t only preserve their line breaks, they also keep all indentation intact relative to their closing set of quotation marks. So in the following case, only our bullet points will end up being indented when our string is rendered:
let infoMessage = """
Welcome to my animation tool. You can use it to:
- Convert images into animations.
- Split an animation up into images.
- Change the frame rate of an animation.
"""
Since multiline string literals are terminated by multiple quotation marks, we can also freely use single quotation marks within them, without requiring any escaping. So even though the following assertion message just has one line of text, it’s still benefitting from being a multiline literal:
assertionFailure("""
An object for the key "\(key)" could not be found.
""")
An alternative to the above approach (besides escaping our quotation marks that is) would be to use a raw string instead.
Finally, we can also use multiline string literals to make a longer inline string a bit easier to read by adding line breaks to it, and by using a backslash (\
) at the end of each line, those line breaks will be ignored when our string is rendered — which can be really useful when writing an error message that we still wish to appear as a single line within our logs:
assertionFailure("""
An object for the key "\(key)" could not be found, \
perhaps you forgot to add it using setObject(forKey:)?
""")
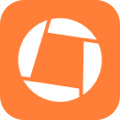
Swift by Sundell is brought to you by the Genius Scan SDK — Add a powerful document scanner to any mobile app, and turn scans into high-quality PDFs with one line of code. Try it today.