This article has been archived, as it was published several years ago, so some of its information might now be outdated. For more recent articles, please visit the main article feed.
How the raw values of Int-based enums get incremented
Basics article available: EnumsBy default, the raw values for an enum backed by Int
(or any other numeric) start at zero, and then get incremented by one for each case that the enum contains. For example, here we’ve defined an Int
-based enum that contains layer indexes for a drawing application, which has the following raw values:
enum LayerIndex: Int {
case shapes // 0
case images // 1
case text // 2
case overlays // 3
}
While the above is certainly a very reasonable default, what if we’d like to start our indexes at a number other than zero? For example, our drawing engine might be treating zero indexes in some special way, or we might want to make our code compatible with another system that uses different numeric conventions.
The good news is that all that we have to do to make that happen, while still enabling the compiler to auto-increment our raw values, is to give our first case a custom value that we’d like to start at — like 1
for instance:
enum LayerIndex: Int {
case shapes = 1 // 1
case images // 2
case text // 3
case overlays // 4
}
The cool thing is that we can keep overriding the raw values for specific cases, and the auto-incrementing process will be adjusted accordingly:
enum LayerIndex: Int {
case shapes = 1 // 1
case images // 2
case text = 9 // 9
case overlays // 10
}
We could, of course, also assign completely custom raw values to every single case, but that’s often harder to maintain — so it’s really neat that we’re still able to take full advantage of the compiler’s auto-incrementing mechanism, even if we want to tweak just a few of our raw values.
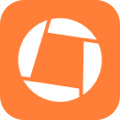
Swift by Sundell is brought to you by the Genius Scan SDK — Add a powerful document scanner to any mobile app, and turn scans into high-quality PDFs with one line of code. Try it today.