This article has been archived, as it was published several years ago, so some of its information might now be outdated. For more recent articles, please visit the main article feed.
Functional networking
Basics article available: NetworkingInstead of complex network managers, or view controllers containing hundreds of lines of networking code, I always try to reduce my networking into functions that I can pass into my view controllers:
// With Futures/Promises:
typealias Loading<T> = () -> Future<T>
// Without Futures/Promises:
typealias Loading<T> = (@escaping (T) -> Void) -> Void
// If we model our networking code using functions, our view
// controllers no longer need to be aware of any networking,
// and can simply call those functions in order to retrieve
// their data:
class ProductViewController: UIViewController {
private let loading: Loading<Product>
init(loading: @escaping Loading<Product>) {
self.loading = loading
super.init(nibName: nil, bundle: nil)
}
func loadProduct() {
loading().observe {
...
}
}
}
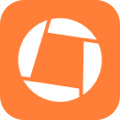
Swift by Sundell is brought to you by the Genius Scan SDK — Add a powerful document scanner to any mobile app, and turn scans into high-quality PDFs with one line of code. Try it today.