This article has been archived, as it was published several years ago, so some of its information might now be outdated. For more recent articles, please visit the main article feed.
Extracting subsequences
Discover page available: The Standard LibrarySwift’s standard library offers several different APIs for extracting a range of elements from any sequence. For example, we can use dropFirst
and dropLast
to remove the first or last element — or use prefix
and suffix
to extract a subsequence with a given amount of leading or trailing elements.
// Since the first argument passed to a command line
// tool is the execution path, we drop it to have the
// first element become the first user-passed argument:
let arguments = CommandLine.arguments.dropFirst()
// Calling 'prefix' or 'suffix' on a sequence lets us
// extract a subsequence containing up to a certain
// number of elements, without crashing if the sequence
// doesn't contain enough elements:
let intro = text.split(separator: " ").prefix(10)
let outro = text.split(separator: " ").suffix(10)
// The above operations are optimized to not cause
// unnecessary copying, so if we want to turn the
// results into a new array, we must do so explicitly:
let words = Array(intro)
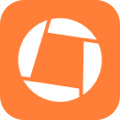
Swift by Sundell is brought to you by the Genius Scan SDK — Add a powerful document scanner to any mobile app, and turn scans into high-quality PDFs with one line of code. Try it today.