Most programming languages ship with some form of standard library that includes built-in, commonly used pieces of functionality, and Swift is no exception. However, Swift takes the concept of a standard library even further, since much of the functionality that we’d perhaps consider to be features baked into the language itself are actually implemented within the standard library.
On this Discover page, you’ll find articles, podcast episodes, tips and tricks that can help you make the most of many of the features and APIs that the standard library offers — which in turn can often help us improve the speed, quality and compatibility of the code that we write.
Collections
Let’s start by taking a look at Swift’s various collection types, and how to perform tasks like sorting, querying, slicing, and transforming elements. We’ll also explore how we can create our very own, custom collections as well.
Article Article Querying collections in various ways
Article Slicing a collection into multiple parts
Article Wrapping collections and other sequences
Article Transforming a collection’s elements
Article What makes Swift’s Set type so powerful
Article How to create custom collections
Article Picking the right data structure for each use case
Strings
Although strings are also technically collections in Swift, they definitely deserve a section of their own. The following articles will give you a thorough look at Swift’s String
type and its various APIs, features, and quirks:
Basics article A basic introduction to Swift strings
Article How Swift’s string API was modernized in Swift 4
Article String parsing in Swift
Tip Character category properties
Tip Converting between String and Data without optionals
Article String literals in Swift
Tip String-based enums in string interpolation
Tip Custom string interpolation
Codable
The built-in Codable
API lets us encode and decode our Swift types to and from various data formats, such as JSON. The fact that Codable
integrates with the Swift compiler itself also means that our implementations of it can often be automatically generated by the compiler — but there are still many different situations in which we might want to tweak things on our own as well. Let’s take a look at how that can be done:
Basics article Let’s start with the basics of Codable
Article Customizing Codable types in Swift
Article Ignoring invalid JSON elements when using Codable
Tip Annotating properties with default decoding values
Tip Testing custom Codable implementations
Article Using Swift’s type inference capabilities with Codable
Next, a quick message from this week’s sponsor
Swift by Sundell is completely free for everyone, but if you’d like to support my work, then please take just a few minutes to check out the following sponsor, since doing so really helps me out financially.
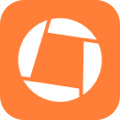
Swift by Sundell is brought to you by the Genius Scan SDK — Add a powerful document scanner to any mobile app, and turn scans into high-quality PDFs with one line of code. Try it today.
Result
Another incredibly convenient type that ships as part of the standard library is Result
, which lets us model the result of an operation as either a success or a failure. Although defining a custom result type takes less than 10 lines of code, the power of having such a type built into the standard library (besides the convenience aspects) is that it lets us use that type as a “bridge” between different APIs, frameworks and code bases — since they can all use the same, default type to model their results.
Errors
The standard library also ships with a dedicated Error
protocol that plays a key part in Swift’s standard error handling system — and making full use of that protocol, and the way it lets us propagate and handle errors, can really make an app feel much more polished and user-friendly.
Podcast conversations about the standard library
The Swift standard library was a key topic on the following podcast episodes, which also contain discussions on how we can write our own code in ways that match the design and overall concepts of the standard library itself:
Other tips and tricks
Finally, let’s wrap up this Discover page with a few tips on how to use the standard library’s randomization functionality, how to extend the standard library in various ways, and a few key APIs that were introduced in Swift 5.1: