This article has been archived, as it was published several years ago, so some of its information might now be outdated. For more recent articles, please visit the main article feed.
The flexible syntax of enums
Basics article available: EnumsOne of the things I love about Swift enums is how flexible the syntax is — both when defining cases and when handling them in a switch statement (which I always recommend, to ”force” the code to handle all various states).
// There are many different ways to define enum cases in Swift:
enum PlaybackState {
// With one or many associated values with explicit labels:
case paused(progress: Percentage)
case playing(progress: Percentage, playbackSpeed: Double)
// Without labels:
case failed(Error)
// Or without any associated values at all:
case finished
}
// Regardless, we have a lot of freedom when choosing how to
// handle a case at the call site:
func updatePlaybackPositionView(for state: PlaybackState) {
switch state {
// We can handle multiple values, and pick which ones we
// want to handle, and which we want to ignore:
case .paused(let progress), .playing(let progress, _):
view.fillRate = progress
// Or simply ignore all associated values if they're not
// relevant for this particular piece of code:
case .failed, .finished:
break
}
}
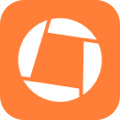
Swift by Sundell is brought to you by the Genius Scan SDK — Add a powerful document scanner to any mobile app, and turn scans into high-quality PDFs with one line of code. Try it today.